Introduction
When working with PrimeVue, creating user-friendly interfaces is a key goal for developers. One common issue that arises is the presence of a caret on popovers, which can detract from the overall aesthetic and user experience of your application. If you’re looking to enhance your PrimeVue popovers by removing the caret, you’ve come to the right place. In this comprehensive guide, we will delve into the reasons you might want to get rid of the caret on popovers in PrimeVue, the steps to achieve this, and best practices for using popovers effectively. Let’s embark on this journey to create cleaner, more elegant user interfaces!
Understanding PrimeVue and Popovers
What is PrimeVue?
PrimeVue is a powerful UI component library for Vue.js, designed to help developers build responsive and visually appealing applications. With a wide range of components, PrimeVue simplifies the process of creating user interfaces, enabling developers to focus more on functionality rather than design.
What are Popovers?
Popovers are a type of overlay that displays additional information when a user interacts with a specific element on the page, such as a button or link. They are commonly used for tooltips, contextual help, or to provide supplementary content without navigating away from the current view.
Why Remove the Caret?
The caret on popovers often indicates the direction of the popover’s content, typically pointing to the element that triggered it. While this can be useful in some contexts, there are several reasons you might want to get rid of the caret on popovers in PrimeVue:
- Aesthetic Preference: For certain designs, a clean look without the caret may be more visually appealing.
- User Experience: In some cases, the caret may confuse users, especially if it misaligns or is inconsistent with the overall design language of your application.
- Customization: Tailoring your popovers to fit your specific design requirements may necessitate removing the caret.
Steps to Get Rid of the Caret on Popovers in PrimeVue
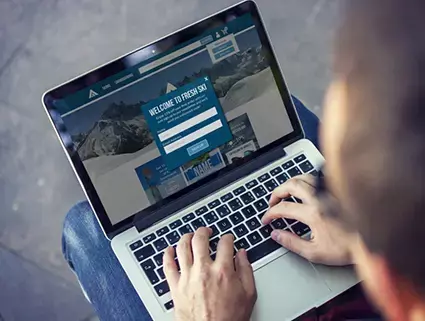
Step 1: Set Up Your PrimeVue Environment
Before diving into the specifics of removing the caret, ensure you have a working PrimeVue setup. If you haven’t already set up PrimeVue, follow these steps:
- Install PrimeVue: Use npm or yarn to install PrimeVue in your Vue project:bashCopy code
npm install primevue --save
- Import Components: Import the necessary PrimeVue components in your main.js or App.vue file:javascriptCopy code
import PrimeVue from 'primevue/config'; import 'primevue/resources/themes/saga-blue/theme.css'; // Choose your theme import 'primevue/resources/primevue.min.css'; import 'primeicons/primeicons.css'; Vue.use(PrimeVue);
Step 2: Create a Basic Popover Component
Create a simple popover component to work with. Here’s an example:
vueCopy code<template>
<div>
<Button label="Show Info" @click="showPopover" />
<Popover v-if="isVisible" :style="popoverStyle" @hide="isVisible = false">
<h3>Popover Title</h3>
<p>This is some information inside the popover.</p>
</Popover>
</div>
</template>
<script>
import { ref } from 'vue';
import { Button } from 'primevue/button';
import { Popover } from 'primevue/popover';
export default {
components: {
Button,
Popover
},
setup() {
const isVisible = ref(false);
const showPopover = () => {
isVisible.value = !isVisible.value;
};
return { isVisible, showPopover };
}
}
</script>
<style>
/* Add custom styles if needed */
</style>
Step 3: Customize the Popover to Remove the Caret
To remove the caret from the popover, you can achieve this through CSS. PrimeVue uses specific classes for its popover components, allowing you to override styles effectively.
1. Identify the Caret Class
Inspect the popover using your browser’s developer tools to identify the class that styles the caret. Typically, it might be something like .p-popover-arrow
.
2. Add Custom CSS
You can then add CSS rules to hide or modify the appearance of the caret. For example:
cssCopy code.p-popover-arrow {
display: none; /* This will completely hide the caret */
}
Alternatively, if you want to keep the space but change its appearance, you could use:
cssCopy code.p-popover-arrow {
width: 0;
height: 0;
border: none; /* This will remove the caret but keep the space */
}
Step 4: Test Your Changes
Once you’ve added your CSS rules, test your application to ensure the caret is no longer visible on the popovers. Interact with the buttons to open and close the popovers, confirming that the changes have taken effect.
Best Practices for Using Popovers in PrimeVue
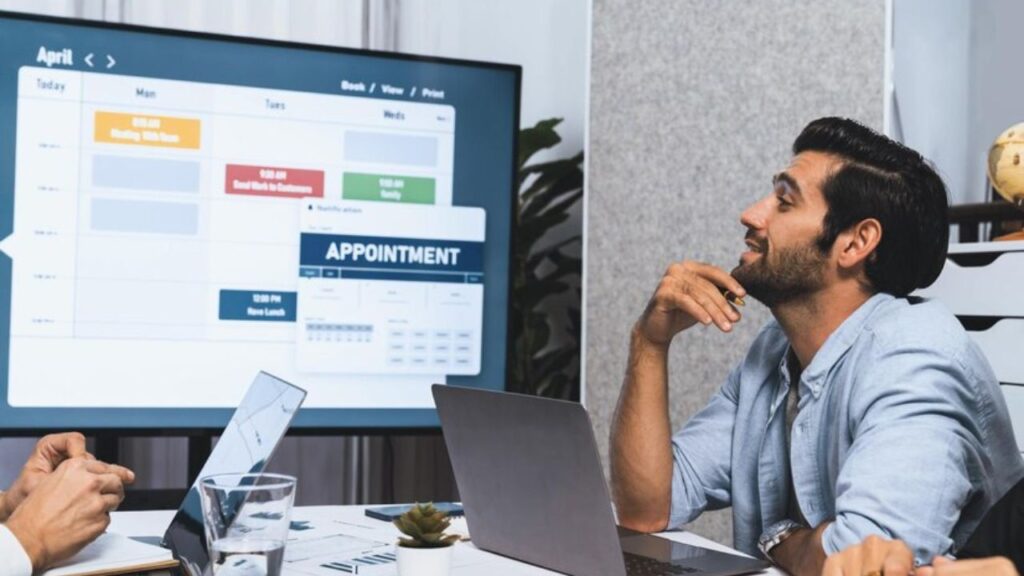
1. Ensure Accessibility
When designing popovers, it’s crucial to ensure they are accessible to all users. Consider the following:
- Keyboard Navigation: Ensure users can navigate to and dismiss the popover using keyboard shortcuts.
- Screen Readers: Provide appropriate ARIA attributes to enhance screen reader compatibility.
2. Keep Content Concise
Popovers should be used for brief information. Avoid overloading them with too much text or complex content. Aim for clarity and brevity.
3. Positioning Matters
Consider the placement of your popovers. Ensure they don’t obstruct important UI elements and are positioned in a way that they are easily noticeable.
4. Test Responsiveness
Test your popovers on various screen sizes and devices. Ensure they function correctly on mobile devices and that the absence of a caret doesn’t confuse users.
Deep Dive into Popover Customization
Understanding Popover Properties
To effectively customize your popover, it’s essential to understand the properties available in PrimeVue’s Popover component:
- visible: A boolean indicating whether the popover is shown.
- style: Custom styles that can be applied directly to the popover.
- appendTo: Controls where the popover will be rendered in the DOM (e.g., ‘body’ or ‘self’).
Customizing Popover Content
Popovers can contain a variety of content types. Here are some examples:
1. Using HTML Content
You can pass HTML content directly into a popover, making it versatile for different use cases:
vueCopy code<Popover>
<template v-slot:content>
<div>
<h3>Detailed Information</h3>
<p>This popover can contain any HTML content you need!</p>
</div>
</template>
</Popover>
2. Integrating Components
You can also integrate other Vue components within your popover for a more dynamic experience:
vueCopy code<Popover>
<template v-slot:content>
<MyCustomComponent />
</template>
</Popover>
Adding Animations
To enhance user experience, consider adding animations when showing or hiding the popover. You can achieve this using CSS transitions:
cssCopy code.p-popover {
transition: opacity 0.3s ease;
}
.p-popover-enter-active,
.p-popover-leave-active {
opacity: 0;
}
Managing Popover Lifecycle
Managing the lifecycle of your popovers is crucial for performance and usability. Here’s how you can handle events like showing and hiding:
vueCopy code<Popover
@show="onShow"
@hide="onHide"
>
<!-- Popover Content -->
</Popover>
javascriptCopy codemethods: {
onShow() {
console.log('Popover is shown');
},
onHide() {
console.log('Popover is hidden');
}
}
Common Use Cases for Popovers
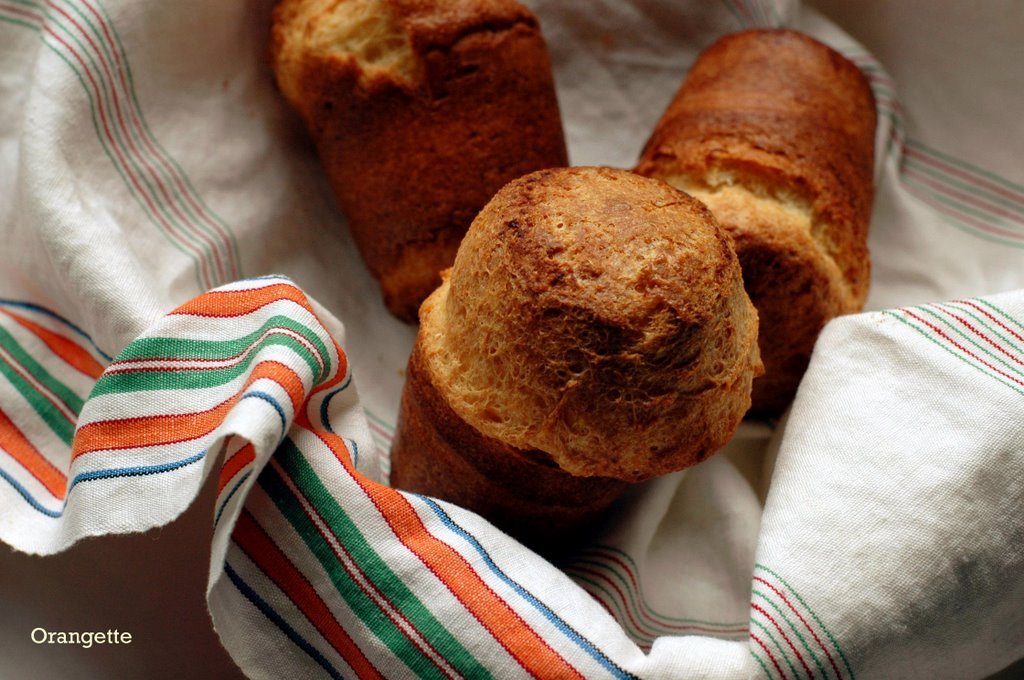
1. Tooltips for Buttons
One of the most common uses of popovers is to provide tooltips for buttons, giving users additional context:
vueCopy code<Button label="Save" @click="save" v-popover="'Click to save your changes'" />
2. Contextual Help
Popovers are excellent for offering contextual help, guiding users through complex forms or applications. This can significantly improve user experience by providing immediate assistance:
vueCopy code<Popover>
<template v-slot:content>
<p>Fill out all fields to proceed. For detailed information, refer to our documentation.</p>
</template>
</Popover>
3. Displaying User Information
If your application includes user profiles, popovers can be a great way to display additional user information when hovering or clicking on user avatars:
vueCopy code<Avatar @click="showPopover = true" />
<Popover v-if="showPopover">
<UserProfile :user="selectedUser" />
</Popover>
Troubleshooting Common Issues
1. Popover Not Displaying
If your popover is not displaying, ensure:
- The component is correctly imported.
- The
visible
property is set correctly. - CSS styles are not hiding the popover unintentionally.
2. Positioning Issues
If the popover appears in an unexpected position:
- Check the
appendTo
property to ensure it’s set according to your needs (e.g., ‘body’ or ‘self’). - Inspect CSS styles that might be affecting positioning.
3. Accessibility Concerns
Make sure you implement ARIA roles and attributes, ensuring that all users