Introduction
When you’re working with websites, especially those built on platforms like Concrete5, you might come across an error that reads: “Call to a member function getCollectionParentID() on null.” This error can seem intimidating at first, but once you understand it, fixing it becomes much easier. Let’s break it down and explore the possible causes, implications, and solutions.
What Does the Error Mean?
At its core, this error message is telling you that your code is trying to perform an action on something that doesn’t exist. Here’s a more detailed breakdown:
“Call to a member function”: This part of the message means that the code is trying to use a specific function or method associated with an object. In programming, objects can have functions that allow you to interact with them in various ways.
“getCollectionParentID()”: This is the specific function that the code is trying to call. It’s designed to retrieve the ID of the parent collection (like a parent page or parent category) of the current object.
“on null”: This indicates that the object the function is supposed to work on is “null.” In simple terms, “null” means “nothing” or “does not exist.” So, the code is trying to retrieve the parent ID of something that doesn’t exist.
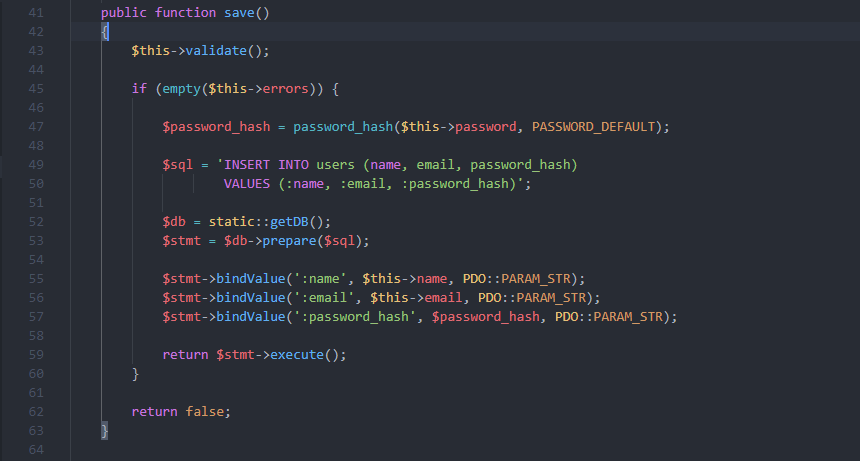
Possible Causes of the Error
This error can occur due to several reasons:
Non-Existent Collection or Page:
The most common reason is that the page, collection, or object the code is trying to interact with doesn’t exist. This could happen if a page was deleted, moved, or never created.
Incorrect Data Handling:
Sometimes, the data passed to your function might not be what you expect. For example, if you’re passing an ID to retrieve a page, and that ID is wrong or points to a non-existent page, the system will return null.
Timing Issues:
In some cases, this error might occur because of a timing issue. For example, the code might try to access a page or collection before it has been fully loaded or initialized, leading to a null reference.
User Permissions:
If your website uses a system where certain pages or collections are restricted based on user roles or permissions, the current user might not have access to the page. As a result, the system might return null when trying to access a restricted collection.
How to Diagnose the Problem
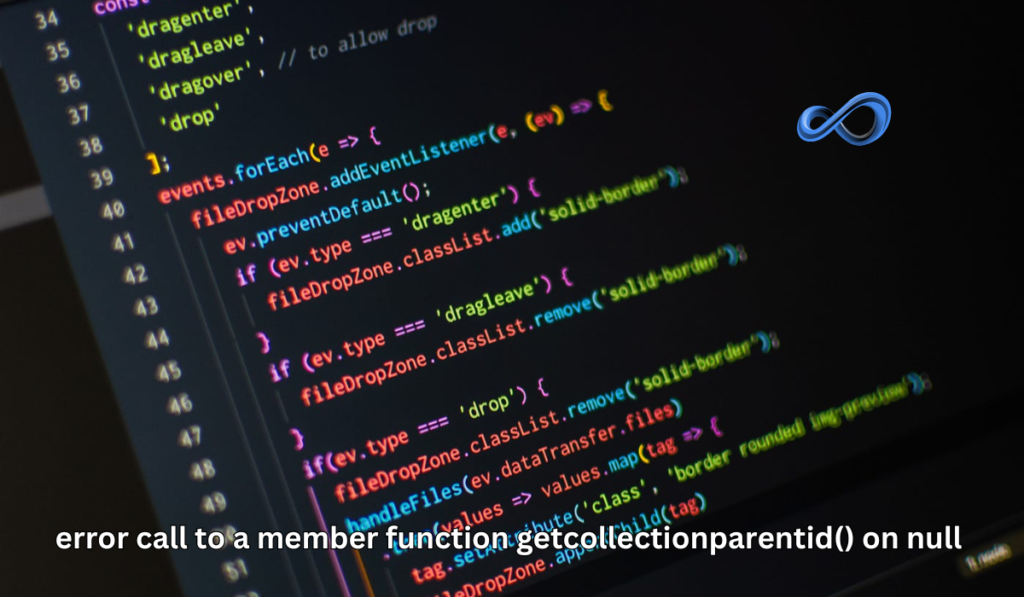
To effectively diagnose and fix this error, you can follow these steps:
Review the Error Log:
Start by looking at the error logs or debugging output. This will give you more context about where the error occurred in your code. Look for the exact line number and the surrounding code.
Check for Null Before Calling the Function:
Before calling getCollectionParentID()
, ensure that the object you’re working with is not null. You can do this by adding a simple check:phpCopy codeif ($collection) { $parentID = $collection->getCollectionParentID(); } else { // Handle the situation where the collection doesn't exist // For example, log an error, redirect to another page, or show a user-friendly message error_log('Collection is null. Cannot retrieve parent ID.'); }
Ensure the Object Exists:
Double-check that the object (such as a page or collection) exists in the system. This might involve checking the database or content management system to ensure that the page ID or collection ID being used is valid.
Check User Permissions:
If your site uses permissions, verify that the current user has the necessary rights to access the page or collection. If permissions are causing the object to be null, you might need to handle this case specifically in your code.
Use Debugging Tools:
Tools like var_dump()
or print_r()
can be useful to print out the contents of variables before the error occurs. This way, you can see exactly what data is being passed and identify why it might be null.phpCopy codevar_dump($collection); if ($collection) { $parentID = $collection->getCollectionParentID(); } else { error_log('Collection is null.'); }
Fallback Mechanism:
Implement a fallback mechanism in case the collection is null. For instance, you can redirect users to a default page or show a message like “Page not found” if the object doesn’t exist.
Preventing the Error in the Future
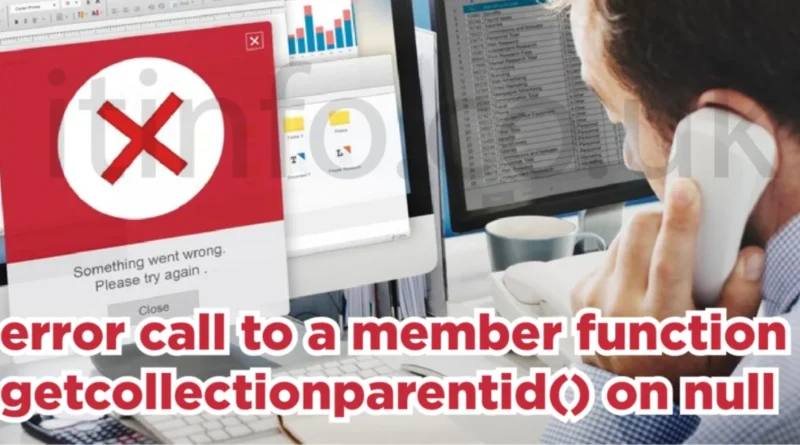
To avoid encountering this error in the future, consider the following practices:
Validation and Checks:
Always validate inputs and check objects before using them. Ensure that objects are properly initialized and exist before calling methods on them.
Error Handling:
Implement robust error handling to gracefully manage cases where data might be missing or null. This will prevent the entire site from crashing and instead provide a user-friendly experience.
Keep the System Updated:
Ensure your CMS, plugins, and custom code are regularly updated. Sometimes, bugs or deprecated functions can lead to such errors, and updates might include fixes.
Proper User Access Management:
Regularly audit user permissions to ensure that users have the correct access rights. Misconfigured permissions can often lead to unexpected null objects.
Case Study: Resolving the “Call to a Member Function getCollectionParentID() on Null” Error
Background
ABC Web Solutions, a small web development company, was hired to maintain and update a website built on Concrete5, a popular content management system (CMS). The website was functioning well, but after making some updates, they encountered a persistent error that read: “Call to a member function getCollectionParentID() on null.”
The Problem
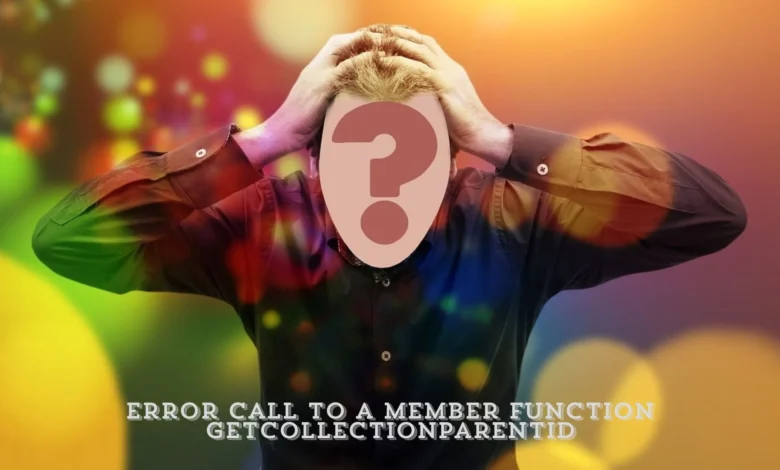
The error appeared whenever a user tried to access a specific section of the website. This section contained important information, and the error made it inaccessible, frustrating both the site’s visitors and the development team.
Investigation
The ABC Web Solutions team began by reviewing the code where the error occurred. They noticed that the function getCollectionParentID()
was being called to retrieve the ID of a parent page. However, the object this function was supposed to work on was returning null, meaning it didn’t exist.
Key Findings
Missing Page: After some investigation, the team discovered that the page the function was trying to access had been accidentally deleted during the update process. This caused the object to be null, triggering the error.
Lack of Error Handling: The code did not include any checks to ensure the page existed before trying to access its parent ID. This lack of error handling meant that when the page was missing, the system couldn’t handle the situation gracefully.
Solution
Restoring the Page: The team quickly restored the missing page from a backup, which immediately resolved the error for users trying to access the site.
Adding Error Handling: To prevent future issues, the team added error handling to the code. They implemented a check to ensure that the page object wasn’t null before calling the getCollectionParentID()
function.Here’s a simplified version of what they added to the code:phpCopy codeif ($collection) { $parentID = $collection->getCollectionParentID(); } else { // Display a friendly message or redirect to a safe page echo "Sorry, the page you are looking for doesn't exist."; }
Outcome
By restoring the missing page and adding proper error handling, ABC Web Solutions not only fixed the immediate issue but also made the website more robust. The site now handles missing pages gracefully, ensuring that visitors don’t see confusing error messages. The client was pleased with the quick resolution and the improved reliability of their website.
Frequently Asked Questions (FAQs)
What does the “Call to a Member Function getCollectionParentID() on Null” error mean?
This error means that the website is trying to use a function on something that doesn’t exist. Specifically, it’s trying to find the parent ID of a page or collection, but that page or collection is missing or wasn’t created properly.
Why did this error happen on my website?
The error usually happens because the page or collection your website is trying to access doesn’t exist. This could be due to accidental deletion, a problem with the code, or an issue with user permissions.
How can I fix this error?
To fix this error, you need to check the part of the code where it’s occurring. Make sure that the page or collection exists. If it doesn’t, you may need to restore it from a backup or handle the situation in the code by checking if the object is null before using it.
Can I prevent this error from happening again?
Yes, you can prevent this error by adding error handling to your code. Always check if the object exists before trying to use it. You should also make sure that pages or collections aren’t accidentally deleted and that user permissions are correctly set up.
What should I do if I don’t understand the error message?
If you’re not familiar with coding, it’s best to consult with a web developer who can help you diagnose and fix the issue. They can look into the specific code causing the problem and implement a solution.
Is this error specific to Concrete5?
While this error message is commonly seen in Concrete5, similar errors can occur in other content management systems or custom-built websites. The underlying issue is the same: the website is trying to use a function on something that doesn’t exist.
What if the error appears after a recent update?
If the error started appearing after an update, it’s possible that the update caused a page or collection to be deleted or that the code was changed in a way that introduced the error. Review the update to see if anything was removed or altered, and consider restoring the site to a previous version if necessary.
Conclusion
The “Call to a member function getCollectionParentID() on null” error occurs when your code tries to use a function on an object that doesn’t exist. This can be caused by non-existent pages, incorrect data handling, timing issues, or user permissions. By understanding the causes and following the steps to diagnose and fix the issue, you can resolve this error effectively and prevent it from happening again. Always ensure your code includes checks for null objects, and handle potential errors gracefully to maintain a smooth user experience.